How do I learn to program?
Saying somone can't learn to tell a computer what to do and how to do it, or learn any other skill for that matter, is selling them (or yourself) short. It's about the right tools, a desire to learn, and setting aside the time to make it happen.
A short question, with a long answer. A decade in, I'm still figuring out the answer.
Are you just curious and want to dabble? Do you want to switch careers? Is it about something (or someone) else? Maybe you've been telling yourself for years that you're not a computer person, but saying you can't learn a skill is selling yourself short. Natural abilities affect the starting line and the pace, but marathons are won with fortitude, time, and patience...... and learning to program well is a marathon.
What is programming?
It's tough to define programming, maybe because it's so vague to begin with.
- For some, it's a means to an end, like automating a tedious task.
- For others, it's a creative pursuit - a game, or expression of art.
- For still others, it's a paycheck. It might be all three, or something else.
If I had to summarize it in one sentence, I think I'd say that:
Programming is writing instructions for a computer, to achieve some end goal beyond what the computer is already capable of.
It's a means to an end, a solution to a problem, an answer to a question. Not every problem or question, but concrete ones. You might find yourself writing a script in Excel to validate data, or an app to manage inventory or track finances. You could help train pilots or work on cybersecurity for the government.
Where do I begin?
That's the million dollar question isn't it? When I first wanted to be a programmer, I started looking through some random c++ source code I found, but that was completely unmotivating. In hindsight, I think my problem was that I had no reason to learn it, beyond wanting to learn it.
If you set out for a nice afternoon stroll, you'll get somewhere, but who knows exactly where.. or when. Set your sites on a specific destination, and you'll find a way to get there, even if it means clawing and scrabbling over rocky terrain.
What's the best way to learn? Sorry to disappoint, but there isn't one! There are plenty of sites and books and programs with their own magic formulas - take it all with a grain of salt. Take what you read here with a grain of salt. No two people have the exact same talents and skills, background and experience, drive and patience, so everyone's bridge looks a little different.
Start with "why"
Come up with a decent why, and the what and how will follow naturally. If you just want to take up writing code as a career, the why might be obvious - to financially support yourself. In that case, you might need a why for the why, like financing a deck or something.
Otherwise, the "why" is the problem you're trying to solve, and the person or people you're solving it before. You don't need a reason to program, but I do my best work when I know exactly what I'm trying to achieve and why I'm doing it.
Learn basic syntax
When my kids were little, they picked up English by being completely immersed in it. It's amazing. It's also pretty inefficient. If every new skill we learned meant being dumped into it without a guide, roadmap, or clue, it'd take forever. After kids pick up a few basics on their own, they need a more formal teaching.
Learn the basic syntax, in any language of your choice. If it's something you're interested in using for a project or pursuing for a career, it'd make sense to use that. Classes and modules, functions and methods, loops and exception handling, primitive types, etc, etc - these are the building blocks, and they're basically the same everywhere.
There are just too many programming languages to learn the detailed syntax about everything, but they all have similarities. One language might call it select
, another map
, and yet another list comprehension, but all you really need to know is that most languages give you a way to transform the items in one list to create a second list. When you need it, you'll know to look for it.
I joined a C# shop earlier this year, but almost immediately found myself doing React development. A Pluralsight course and a few tutorials over the course of a week, and I was off. There's been constant learning since then, but the basics are the basics.
Learn basic tools
There's a basic set of tools every developer needs, and then some more specialized stuff depending on what kind of work you're doing.
Source code editors
Find an editor that supports a wide array of programming languages, ideally with support for extensions that extend its functionality. VS Code is my favorite right now, but other popular ones include Sublime, Atom, and Notepad++.
Pick one, try it on, feel it out. Some are more powerful than others, but the added complexity can make them more difficult to learn.
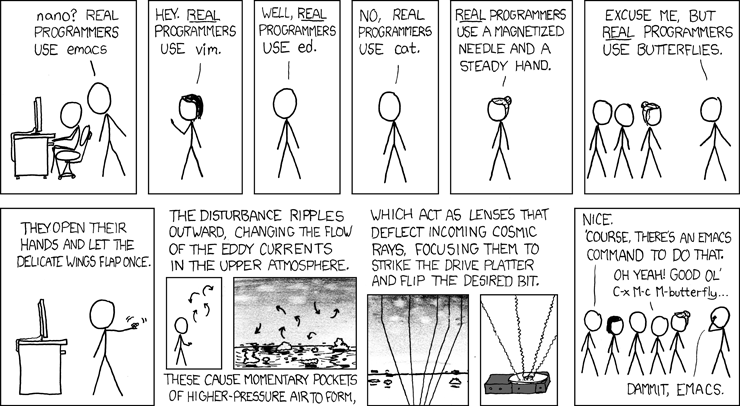
IDEs
An integrated development environment, or IDE, is usually more powerful than a code editor, but only for a specific language or framework. If you're interested in working in a single language, taking the time to find and learn to use an IDE will pay off huge dividends.
- C# has Visual Studio
- Ruby has RubyMine
- Python has PyCharm
- Java has Eclipse and NetBeans
Git / GitHub
Once you start programming and get something working, you'll want to save your progress before making changes. If you mess something up, you'll want a way to go back. The best way to do that used to be copying the source code to another folder. If you do that now, the code gods will curse you and your children so.. don't do that.
Most projects you work on - your own, open source, with teams of people - will end up in source control. The defacto version control standard is git, and the defacto product for using git is GitHub. Get familiar with it.
Testing
If you write a piece of code and you know it works, prove it! Writing tests is a skill that, for some reason, quite a few developers still don't use. Sometimes it's a limitation of the system - old codebases are difficult to wield in a way that supports testing. Sometimes it's a limitation of time - when I'm under the gun on a tight deadline, testing might take a backseat to shippable code.
Familiarize yourself with different types of testing - unit testing, acceptance testing, integration testing, etc. The more types of automated tests you write for a given piece of code, the more sure you can be that it won't unknowingly break on you.
Write some code
You can't learn about syntax and how to use tools without writing a little code, but eventually you're gonna be ready for something.. bigger. But what? Well, what drove you to learn programming in the first place?
If you don't have a project in mind, you could look for an online challenge or jump into an open source project. Writing a browser plugin might be more your style.
Be patient
Writing code is easy. Learning to write code is not. Understanding the simple example above means learning about markup language, event handlers, and some basic JavaScript. There may be times you'll wonder whether you really know anything, or at least whether you know the right stuff.
It'll take much longer than 21 days to become an expert.
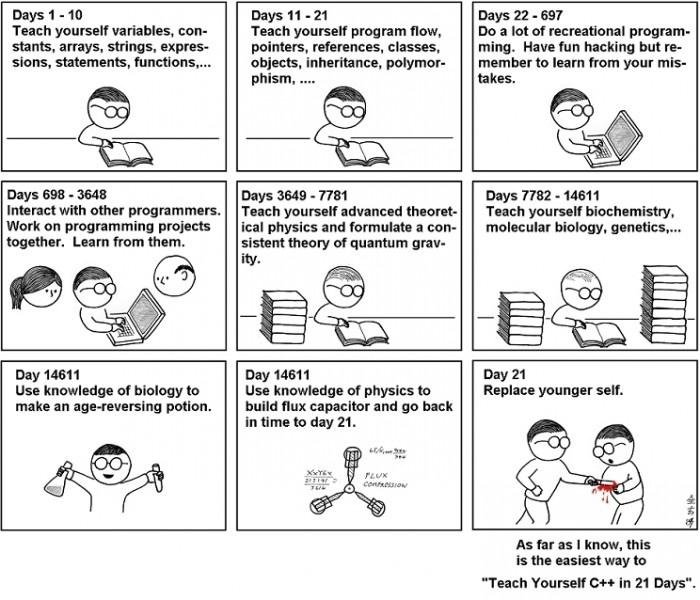
We're always in between what we do know and what we'd like to know, and both sides are growing all the time. There's always going to be a new language, framework, or methodology to learn (especially with JavaScript!), but the fundamentals don't change much.
Take a step back once in awhile to think about what you've accomplished, so you don't get overwhelmed with what you want to learn. Maybe you want to write about your accomplishments in a blog, a private journal, or just find someone to talk to.
Break down the problem
I've been writing code for awhile now, and I still get overwhelmed with projects that are out of my depth. I've gotten better at catching myself staring at the screen with a vague sense of "where the @#$% do I begin??", and realizing when I need to break down the problem.
It doesn't matter if you're working on a huge app with a team at a large company, or your own project in your spare time. Break down what you're trying to do into smaller steps, then rinse and repeat until you feel like you've got something you can actually dig into and start attacking.
If you can't get there, well.. that tells you something too. Maybe you're not exactly sure what the problem is, or what the required solution should look like.
- Can you describe the problem in a single sentence, or a few?
- Can you describe what a solution might look like in a few sentences? How would it fix the problem? How do you envision someone using it?
Don't worry about the details at first - just think about where you'd like to end up, then break the 50 mile journey into short jogs, then individual steps.
Pick a direction and run
Doing isn't frustrating - sitting on the sideline, thinking about all the possibilities, and trying to figure out the place to start is. Sometimes it's better to do anything that achieves your goal, then keep finding better ways to do it.
You'll get better and better over time, but you're never really done!
Comments / Reactions