What is an API?
An API is an Application Programming Interface, but what's that really mean? In a more practical sense, it's one programmer hiding the (possibly messy) details of their own code behind a nice veneer, in order to make it easier for another programmer to consume it in their own program.
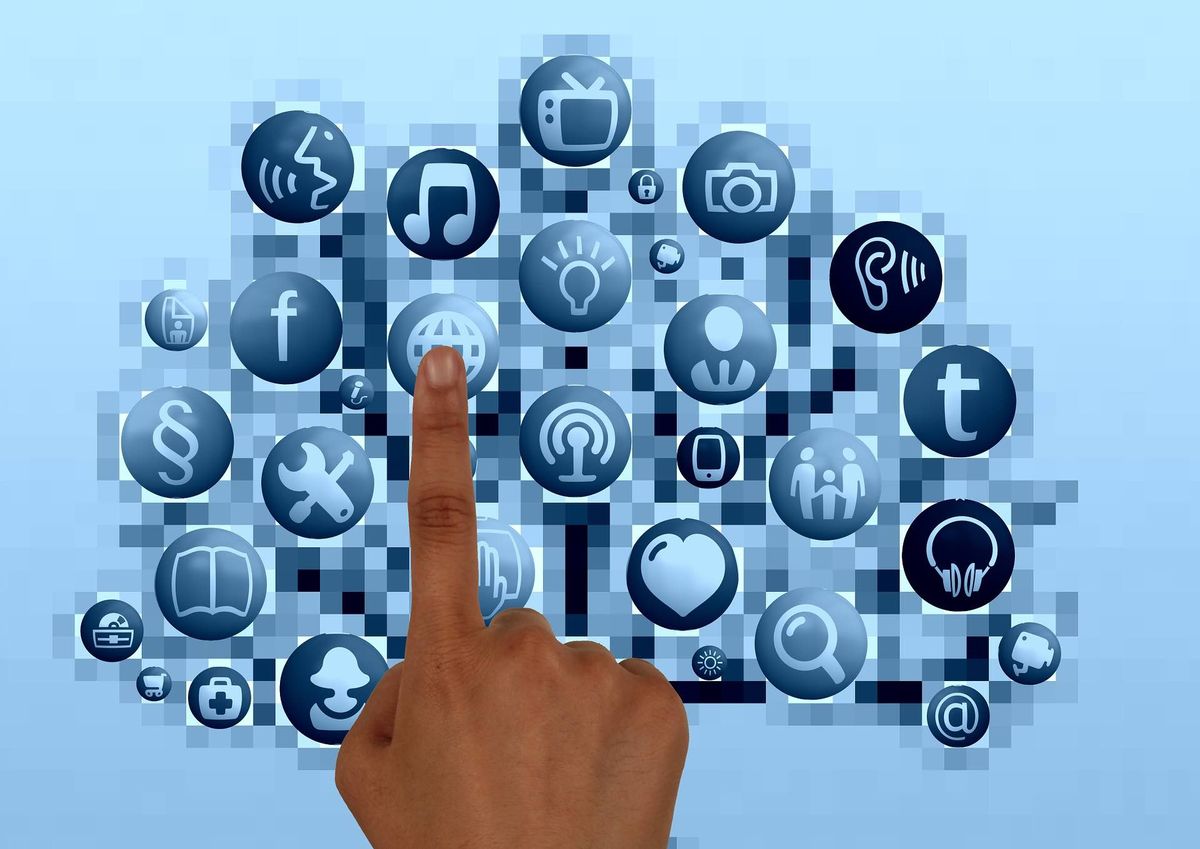
To define it, an API is an Application Programming Interface, but what's that really mean? In a more practical sense, it's one programmer hiding the (possibly messy) details of their own code behind a nice veneer, in order to make it easier for another programmer to consume it in their own program. 😉
First, what's an interface?
Before we talk about programming, just what is an interface in the most general sense? We're absolutely surrounded by them, and may not even realize it most of the time. In fact, the better the interface the less aware we are that it even exists! Any time something complex is abstracted away for us, hiding the complex details behind a little display screen or a simple button push, someone took the time to create an interface for us.
The dashboard in your car is a good example. There's an interface that just returns some information to you, like running a report. You don't have to count the number of times the crankshaft in your engine turns - just glance at the RPM gauge. Better yet, you don't have to remember whether 2000 or 6000 RPM in a certain gear is good or bad - areas of the gauge are color-coded. You don't have to manually inspect the level of gas in the tank, or read the output of the low-fuel sensor - you get a nice readout on the dash and a dedicated indicator light. The dashboard is an interface, abstracting away the inner-workings of dozens of sensors all over the car.
Your kitchen's full of interfaces too, from the toaster to the microwave to the coffee machine. All of these devices usually take little more than one or two button pushes to set off a complex chain of internal motion that we don't need to worry about. Could you imagine if you had to keep glancing inside your toaster and unplug it when your bread was perfectly toasted? Or keep running down to the basement to flip the furnace on and off? It'd be ridiculous!
What's it have to do with programming?
Programming languages have their own interfaces that provide layers of abstraction too. Code is often organized into classes and modules, and then select pieces of the code are marked as "public" or "exported" to let you know those are the ones that are safe to use.
Take a simple "Person" class for example (in C#). Imagine there's a "PersonReport" class that can answer questions regarding a collection of people, like "who's an adult?" or "who's a parent?".
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
public List<Person> Children { get; set; }
}
public class PersonReport
{
public List<Person> GetAdults(List<Person> persons)
{
return persons.Where(x => x.Age >= 18).ToList();
}
public List<Person> GetParents(List<Person> persons)
{
return persons.Where(x => x.Children.Count > 0).ToList();
}
}
Similar to the dashboard in our car, we don't have to worry about the details underneath. In this case the "messy details" is a simple one-liner of LINQ, but it could be many lines calling other methods and other classes - the point is, we don't care. The details are abstracted away behind a clean interface. We just ask for parents and get the results.
So.. what is an API then?
Well, that... and more. Let's consider some code written by another developer (maybe another team within a large corporation, but probably a completely different entity or company) and provided to us as a framework or library. Nearly every language has an existing framework. These are the building-blocks of the language, as well as thousands or even millions of lines of code that prevent you and I from having to reinvent the same wheel over and over.
- In C#, it's the .NET Framework developed by Microsoft.
- In Erlang, it's the OTP libraries from Ericsson.
- Java has its base libraries and a bunch of other frameworks.
- ... and on and on
In the sample of code above, even the call to persons.Where(x => x.Children.Count > 0).ToList();
involved an API call, in the sense that I don't have to know or care how the LINQ library manages to filter my list of persons by a particular attribute. I just specify the condition on which to filter, and call the Where()
function to do its magic.
API calls in the cloud, and the REST interface
So far I've been talking about frameworks and libraries which are normally copied to your machine locally and compiled or interpreted with the rest of your application. But what if the code is in the cloud? The "cloud" is of course just a fun marketing term. We're still talking about code, but it's on someone else's computer instead of yours - could be a state-of-the-art facility or one person working out of their basement. So if you don't have access to the code to compile it into your own, how do you safely access it?
This is the what I think most people mean by APIs now, at least most of the time. There are thousands of services all over the world, written in any one (or a combination) of a hundred different languages, and the problem is how to share all of that with whatever you're writing in the language of your choice? There are a number of ways, but the most popular right now is the REST interface.
Time to back up again for a minute.
Any time you access a page in your web browser, it sends a "GET" message to the page's url like (i.e. "http://www.example.com"), and in return some web server somewhere in the world (you don't care where or how) returns a block of html markup representing that page to you. Your browser uses that and says "okay, there's a table tag so let's add a table, and a blockquote tag so let's format that however it's supposed to look, and oh! an image tag". It renders the page and does more "GET" operations to get all the images and so on and so forth.
And if you fill out a form to register for a site, your browser sends a "POST" (or maybe a "PUT") message to some url specified by the form like "http://www.example.com/registration" and sends all the information you filled out like your name, age, etc. And if you're on a page that lets you view some data and delete some of it, then maybe when you click a little trash icon it sends a "DELETE" message to a particular url along with your user id, and that deletes some resource on their server.
If you want to see something kind of neat, download Postman and try performing a "GET" on some websites. You'll see the markup your browser gets when it requests a site, and you can even use this tool to send "POST" and "DELETE" requests to sites too, if you know of a URL that expects them.
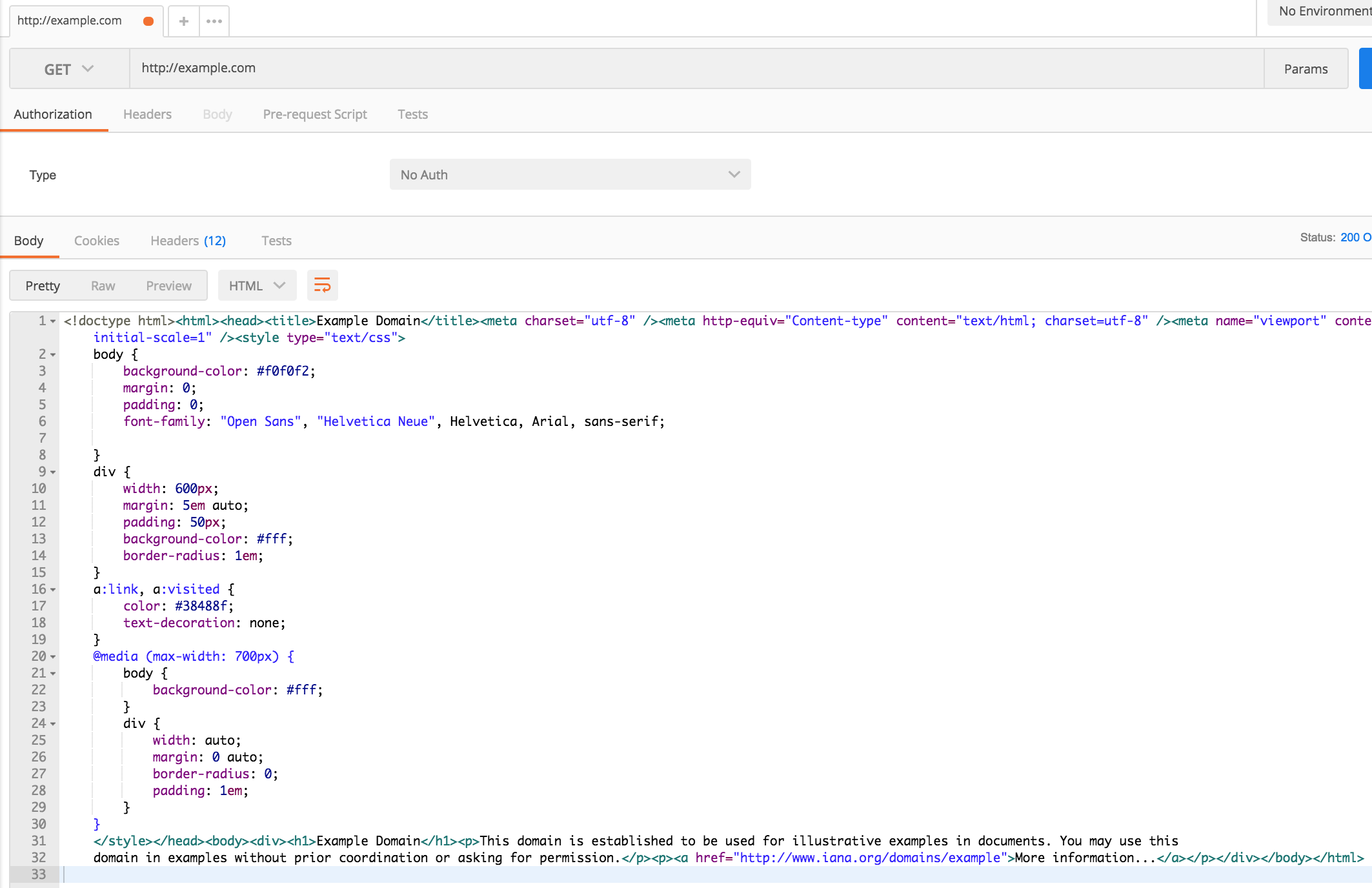
I don't want to get too deep into how REST works, but that's an overview. And if it works for a browser, it can work for us too. Our programs can use the same idea to create and update, get or delete, all kinds of data from someone else's program "out there in the cloud". Your program running on your local machine can call out and do a "GET" to a certain endpoint (url) such as "http://www.example.com/users/1234", and the other server can process that request and say "okay they want the 'users' endpoint which means i should execute method xyz (we don't care what) and get the data for user 1234 and return something back". The difference is that instead of returning html markup, it'll return your data in JSON format... something else that's too big to get into in this post (it's already long enough!).
So all that to say, if someone asks you what an API is...
An API is some code running on someone else's machine (where? how? who cares!), which they've made accessible to you. And the way you typically access it is using REST, very similar to how your web browser does it!
Have you used APIs before? If not, did this help clarify the concept or just muddy the waters? If you have used APIs, how would you define them? Share below!
Comments / Reactions