How to make all images in a Ghost post auto-link to themselves (to view at full resolution)
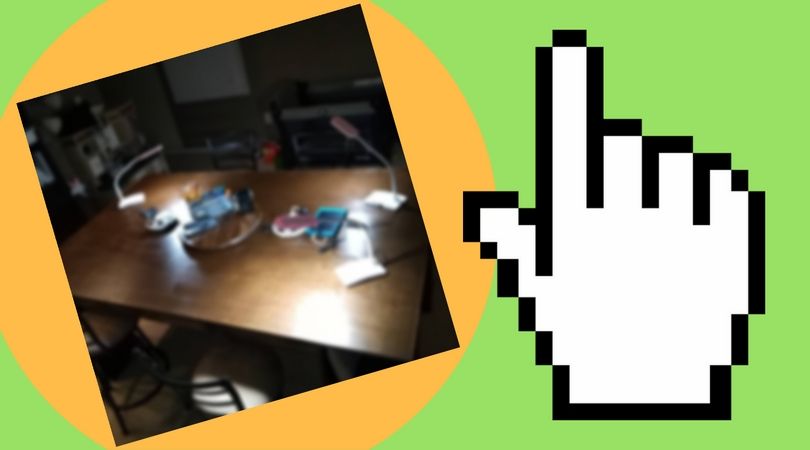
When you insert an image into a post using the Ghost platform, it might be resized (depending on the theme you're using) to a significantly smaller size than its actual dimensions. Viewing the full version means either your visitor has to open the image in a new tab, or you could link the image to itself so that clicking on it opens it up outside of the context of the page - either way, someone's doing manual work. Let's automate this!
Here's a snippet of JavaScript code you can insert under "Blog Header" in the "Code injection" section of your Ghost blog.
<script type="text/javascript">
document.addEventListener("DOMContentLoaded", function(event) {
document.querySelectorAll('.post-content img').forEach(function(image) {
image.style.cursor = "zoom-in";
if (image.title === '') {
image.title += "(click to zoom)"
} else {
image.title += " (click to zoom)"
}
image.onclick = function(event) {
window.location.href = image.src;
};
});
});
</script>
What's it doing?
It runs when the DOMContentLoaded event fires, which occurs after the HTML is rendered, but before any images, stylesheets, etc are downloaded. So we can be sure the image placeholders are present, even if the images themselves are not yet.
It selects all image elements under the element that has the .post-content
class applied to it, which is the main body of your post's content. You probably don't want to apply this script to every image on your site, but you can change change the selector as needed. Read more about querySelectorAll and selectors at MDN.
It loops through each image, doing three things:
- The cursor is changed to "zoom-in", signaling that an action can be taken.
- The title is changed, again to signal that an action can be taken.
- A click event is assigned, that navigates to the image's source.
Comments / Reactions